COBRApy: Constraint-based metabolic modeling in Python#
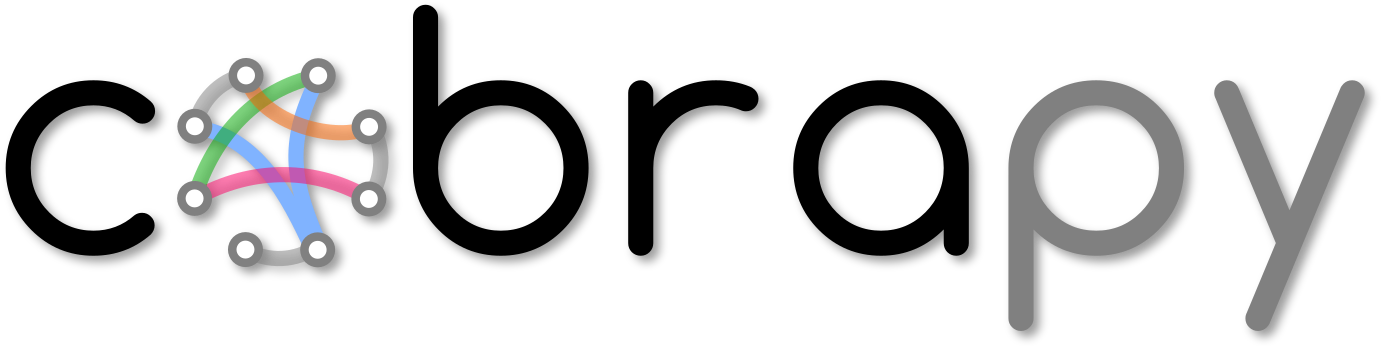
COBRApy is a python package for large-scale analysis of metabolic systems. It offers tools for frequently used COBRA procedures, including flux balance assessment, flux variability studies, and gene removal evaluations.
import corneto as cn
cn.info()
|
|
Genome scale metabolic networks can be customized with COBRApy, and imported to CORNETO for more advanced analysis that require modified versions of methods implemented in the package.
from cobra.io import load_model
model = load_model("textbook")
len(model.metabolites), len(model.reactions)
(72, 95)
from corneto.io import cobra_model_to_graph
G = cobra_model_to_graph(model)
G.shape
(72, 95)
G.get_edge(0), G.get_attr_edge(0)
((frozenset({'acald_c', 'coa_c', 'nad_c'}),
frozenset({'accoa_c', 'h_c', 'nadh_c'})),
{'__edge_type': 'directed',
'id': 'ACALD',
'__source_attr': {'acald_c': {'__value': np.float64(-1.0)},
'coa_c': {'__value': np.float64(-1.0)},
'nad_c': {'__value': np.float64(-1.0)}},
'__target_attr': {'accoa_c': {'__value': np.float64(1.0)},
'h_c': {'__value': np.float64(1.0)},
'nadh_c': {'__value': np.float64(1.0)}},
'default_lb': np.float64(-1000.0),
'default_ub': np.float64(1000.0),
'GPR': 'b0351 or b1241'})
Flux Balance Analysis with COBRA and CORNETO#
Using CORNETO#
CORNETO has the same type of interface for all methods, and FBA can be seen as a special type of network inference method, where we calculate obtain metabolic fluxes for edges. In its basic form, we need to indicate which reaction we want to optimize (maximize its flux) subject to metabolic constraints. For simplicity, here we maximize the biomass reaction of the textbook model from cobrapy
without modifying any default constraint in the network
# This is the id of the biomass reaction
list(G.get_edges_by_attr("id", "Biomass_Ecoli_core"))
[12]
from corneto.methods.future.fba import MultiSampleFBA
d = cn.Data.from_cdict(
{
"fba_example": {
"Biomass_Ecoli_core": {
"role": "objective"
}
}
}
)
m = MultiSampleFBA()
P = m.build(G, d)
# Variables created.
P.expr
{'_flow': _flow: Variable((95,), _flow),
'edge_has_flux': edge_has_flux: Variable((95,), edge_has_flux, boolean=True),
'flow': _flow: Variable((95,), _flow)}
P.solve(solver="scipy")
P.expr.flow[12].value
np.float64(0.873921506968431)
import pandas as pd
pd.DataFrame(P.expr.flow.value, index=G.get_attr_from_edges("id"), columns=["fluxes"])
fluxes | |
---|---|
ACALD | -0.000000 |
ACALDt | 0.000000 |
ACKr | 0.000000 |
ACONTa | 6.007250 |
ACONTb | 6.007250 |
... | ... |
TALA | 1.496984 |
THD2 | 0.000000 |
TKT1 | 1.496984 |
TKT2 | 1.181498 |
TPI | 7.477382 |
95 rows × 1 columns
Using COBRA#
result = model.optimize()
result
fluxes | reduced_costs | |
---|---|---|
ACALD | 0.000000e+00 | 0.000000e+00 |
ACALDt | 0.000000e+00 | 0.000000e+00 |
ACKr | 3.192215e-14 | -0.000000e+00 |
ACONTa | 6.007250e+00 | 0.000000e+00 |
ACONTb | 6.007250e+00 | 1.082023e-16 |
... | ... | ... |
TALA | 1.496984e+00 | -4.759755e-17 |
THD2 | 0.000000e+00 | -2.546243e-03 |
TKT1 | 1.496984e+00 | 0.000000e+00 |
TKT2 | 1.181498e+00 | 2.379877e-17 |
TPI | 7.477382e+00 | 2.316332e-17 |
95 rows × 2 columns
result.to_frame().iloc[12,:]
fluxes 8.739215e-01
reduced_costs 1.561251e-16
Name: Biomass_Ecoli_core, dtype: float64